I previous blogged how to update an InfoPath XML field. There are some
exceptions to when it comes to People Picker fields though. These fields are
stored as complex types and as such, we have to break them down into their
individual sub types and update those types separately in order to the update to
stick. The DisplayName and AccountId fields need to be updated together in this
case. A good example could be when Cindy is transferred from the Help Desk
position to the Marketing Department. There are several tickets in the Help
Desk system that she is the Owner of, but those won't be handled by her any
longer. Those will now be handled by Anne who was hired to fill the open
position. In order to automate the transfer of tickets, we need to have a
programmatic way to change all tickets belonging to Cindy, to now belong to
Anne. Here's how we'll go about it:
SPSite sps = new SPSite(tbl["-url"].ToString()); SPWeb spw = sps.OpenWeb(); SPList lst = spw.Lists[tbl["-library"].ToString()]; SPFile spf; XmlDocument doc; XmlNamespaceManager nsm; int intProcessed = 0, intError = 0; foreach (SPListItem itm in lst.Items) { try { spf = itm.File; doc = new XmlDocument(); doc.Load(spf.OpenBinaryStream()); nsm = new XmlNamespaceManager(doc.NameTable); foreach (XmlAttribute att in doc.DocumentElement.Attributes) { if (att.Prefix == "xmlns") { nsm.AddNamespace(att.LocalName, att.Value); } } if (doc.SelectSingleNode("//my:" + tbl["-field"].ToString() + "/pc:Person/pc:AccountId", nsm).InnerText == tbl["-oldaccountid"].ToString()) { spf.CheckOut(); doc.SelectSingleNode("//my:" + tbl["-field"].ToString() + "/pc:Person/pc:AccountId", nsm).InnerText = tbl["-newaccountid"].ToString(); doc.SelectSingleNode("//my:" + tbl["-field"].ToString() + "/pc:Person/pc:DisplayName", nsm).InnerText = tbl["-newdisplayname"].ToString(); spf.SaveBinary(new MemoryStream(Encoding.UTF8.GetBytes(doc.OuterXml))); spf.CheckIn("Updated by the UpdateInfoPathItems utility!"); } intProcessed++; } catch (Exception ex) { Core.Log(" >>> " + itm.Url, sw, ConsoleColor.Red); Core.Log(" >>> " + ex.Message, sw, ConsoleColor.Red); intError++; } }
The above code references a tbl[] hash table of command line parameters that were parsed out by the Quix Utilities' HashArgs command thus:
System.Collections.Hashtable tbl = Core.HashArgs(args);
Happy coding!
Later
C






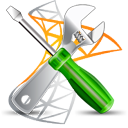

No comments:
Post a Comment
Comments are moderated only for the purpose of keeping pesky spammers at bay.